
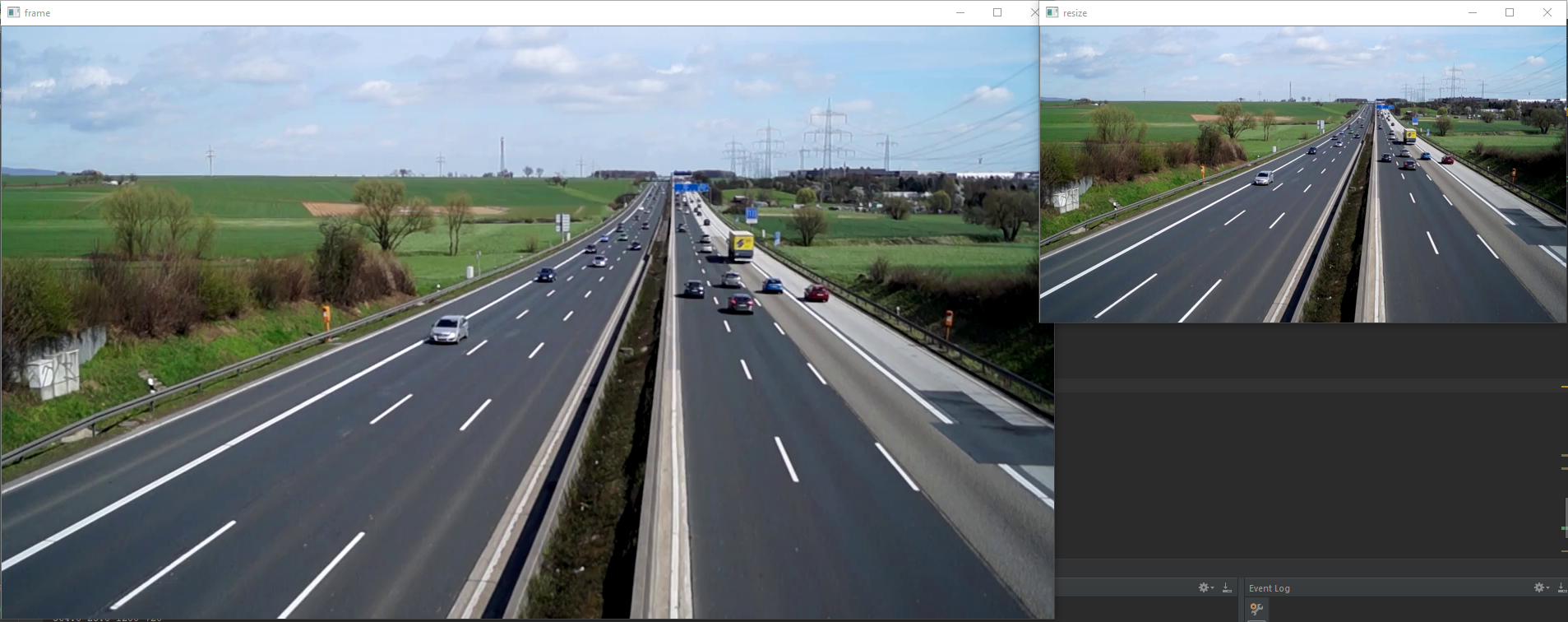
The script is straightforward and can be viewed below or here.
#RESIZE IMAGE OPENCV INSTALL#
This can be done with: “pip install opencv-python” or “conda install -c conda-forge opencv” (whichever package manager you prefer). Batch resize and pad images using Python and OpenCV Batch resize and pad imagesįirst you need to install OpenCV. I chose to use OpenCV because it is a widely-used, easy-to-use library with powerful capabilities. To automate this process for a large number of images, I wrote a Python script using OpenCV. However, this results in a size that is not a multiple of 64, so the image needs to be padded to 1536×512 in order to be processed by the model. If you have an image that is not a multiple of 64, like 599×205 pixels, you can maintain the aspect ratio by resizing it to 1496×512. Stable Diffusion (at least 1.5) works best with images of 512 pixels in width or height. In case of Stable Diffusion, multiples of 64 are required. Just for fun, we crop to get the Thanos’s gauntlet.When using AI models like Stable Diffusion, sometimes input images need to be of a specific size. The first line returns that part of the image which starts and ends between (beginX:endX), (beginY,endY). The output is:Ĭropping of an image in Python cropped_image = image Later the transformation is done by cv2.warpAffine() function whose parameters are the original_image, obtained matrix and the dimension of the rotated image. The parameters are center, angle_of_rotation(here we are rotating for an angle of 180 degrees) and scaling factor. The function cv2.RotationMatrix2D returns a matrix that contains the image of rotated coordinates. Rotated_image = cv2.warpAffine(image, Matrix, (w1, h1))Īs we know now image.shape() returns a tuple up to indexing 2 where the first two values are height and width hence the first line extracts the values of height and width, We then discover the center coordinates by moving towards half of both height and width. Matrix = cv2.getRotationMatrix2D(center, 180, 1.0) Rotation of an image in OpenCV (h1, w1) = image.shape For time being we are not going to focus on the algorithm but sticking to the implementation part. The parameters are the original image, dimension, and the algorithm to be used for this purpose. The third line uses the function cv2.resize() which actually does the main work of changing the size. Both values are then inserted into the variable called dim(dimension of new image).
#RESIZE IMAGE OPENCV CODE#
Resized_image = cv2.resize(image, dimension, interpolation = cv2.INTER_AREA)Īs seen in code the height and width are specified as 300. Resizing of an image in Python with OpenCV h1=300 The values are (height, width, channel) where the channel is the RGB components. The output will be as: (175, 289, 3) as displayed for my image. Normally, the dimensions are width*height but OpenCV takes as height*width. Printing the dimensions of an image in OpenCV print(image.shape)

Reading and displaying of an image – OpenCV Python image=cv2.imread('Thanos.jpg')Ĭv2.imread() is a function which takes image name as a parameter(provided the image is saved in the same folder where the program is or else just give the path to the image) and cv2.imshow() display the image with name and its parameters are (‘name_of_the_window’,variable_in_which_image _is_stored).
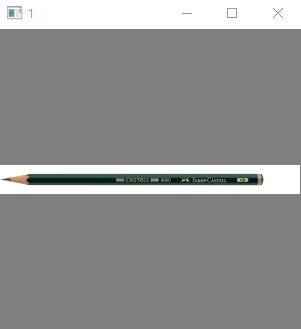
The various operation goes as follows: Importing the required libraries required for the program import cv2Ĭv2 is the OpenCV library and the numpy library is included as many times the image will be treated as an array.
#RESIZE IMAGE OPENCV MOVIE#
To make this session interactive image of Thanos(A character from a famous movie ) is being taken for example. Here we’ll be doing many operations on an image using OpenCV functions.
